c#正则表达式如何使用?代码举例?
C#中使用正则表达式的方法有两种:一是使用System.Text.RegularExpressions命名空间中的类;二是使用支持正则表达式的String类的方法。
以下是使用System.Text.RegularExpressions命名空间中的类来匹配一个字符串的示例代码:
string inputString = "Hello World";
Regex reg = new Regex(@"bHellob");
Match match = reg.Match(inputString);
if (match.Success) {
Console.WriteLine("Match found!");
}
C#中的Regex定义了一个正则表达式。Regex类提供了解析大型文本以查找字符模式的方法和属性。在本文中,您将学习如何在C#中使用Regex类。
正则表达式用于检查字符串是否与模式匹配。c# regex也称为c#正则表达式或c# regexp是定义模式的字符序列。模式可以由文字、数字、字符、操作符或结构组成。该模式用于搜索字符串或文件,以查看是否找到匹配项。正则表达式经常用于输入验证、解析和查找字符串。例如,检查有效的出生日期、社会保险号、全名(名字和姓氏用逗号分隔)、查找子字符串的出现次数、替换子字符串、日期格式、有效的电子邮件格式、货币格式,等等。
1. C# Regex类
C# Regex类表示正则表达式引擎。它可以用来快速解析大量的文本以找到特定的字符模式;提取、编辑、替换或删除文本子字符串;并将提取的字符串添加到集合中以生成报告。
Regex类定义在System.Text。RegularExpressions名称空间。Regex类构造函数将模式字符串作为参数,并带有其他可选参数。
下面的代码片段从一个模式创建了一个正则表达式。这里的模式是匹配以字符' M '开头的单词。
// Create a pattern for a word that starts with letter "M"
string pattern = @"b[M]w+";
// Create a Regex
Regex rg = new Regex(pattern);
下面的代码片段有一个很长的文本,其中包含需要解析的作者名称。
// Long string
string authors = "Mahesh Chand, Raj Kumar, Mike Gold, Allen O'Neill, Marshal Troll";
Matches方法用于查找正则表达式中的所有匹配项,并返回MatchCollection。
// Get all matches
MatchCollection matchedAuthors = rg.Matches(authors);
下面的代码片段循环遍历matches集合。
// Print all matched authors
for (int count = 0; count < matchedAuthors.Count; count++)
Console.WriteLine(matchedAuthors[count].Value);
下面是完整的代码:
// Create a pattern for a word that starts with letter "M"
string pattern = @"b[M]w+";
// Create a Regex
Regex rg = new Regex(pattern);
// Long string
string authors = "Mahesh Chand, Raj Kumar, Mike Gold, Allen O'Neill, Marshal Troll";
// Get all matches
MatchCollection matchedAuthors = rg.Matches(authors);
// Print all matched authors
for (int count = 0; count < matchedAuthors.Count; count++)
Console.WriteLine(matchedAuthors[count].Value);
在上面的例子中,代码寻找字符 ' M '。但是如果单词以“m”开头呢?下面的代码片段使用了RegexOptions。IgnoreCase参数,以确保Regex不查找大写或小写。
// Create a pattern for a word that starts with letter "M"
string pattern = @"b[m]w+";
// Create a Regex
Regex rg = new Regex(pattern, RegexOptions.IgnoreCase);
2. 使用Regex替换多个空格
Regex.Replace()方法的作用是:将一个匹配的字符串替换为一个新的字符串。下面的示例在字符串中查找多个空格,并用单个空格替换。
// A long string with ton of white spaces
string badString = "Here is a strig with ton of white space." ;
string CleanedString = Regex.Replace(badString, "\s+", " ");
Console.WriteLine($"Cleaned String: {CleanedString}");
下面的代码片段将空格替换为' - '。
string CleanedString = Regex.Replace(badString, "\s+", "-");
3.在C#中使用Regex替换多个空白
下面的示例使用正则表达式模式[a-z]+和Regex.Split()方法拆分任意大写或小写字母字符的字符串。
// Spilt a string on alphabetic character
string azpattern = "[a-z]+";
string str = "Asd2323b0900c1234Def5678Ghi9012Jklm";
string[] result = Regex.Split(str, azpattern,
RegexOptions.IgnoreCase, TimeSpan.FromMilliseconds(500));
for (int i = 0; i < result.Length; i++)
{
Console.Write("'{0}'", result[i]);
if (i < result.Length - 1)
Console.Write(", ");
}
正则表达式是用于字符串解析和替换的模式匹配标准,是计算机用户用来表示计算机程序应该如何在文本中查找指定的模式,以及在找到每个模式匹配时程序应该做什么的一种方式。有时它被缩写为“regex”。它们是查找和替换采用已定义格式的字符串的强大方法。
下面是一个用C#编写的简单代码示例,展示了如何使用正则表达式。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Text.RegularExpressions;
namespace RegularExpression1
{
class Program
{
static void Main(string[] args)
{
Regex r = new Regex(@"^+?d{0,2}-?d{4,5}-?d{5,6}");
//class Regex Repesents an immutable regular expression.
string[] str = { "+91-9678967101", "9678967101", "+91-9678-967101", "+91-96789-67101", "+919678967101" };
//Input strings for Match valid mobile number.
foreach (string s in str)
{
Console.WriteLine("{0} {1} a valid mobile number.", s,
r.IsMatch(s) ? "is" : "is not");
//The IsMatch method is used to validate a string or
//to ensure that a string conforms to a particular pattern.
}
}
}
}
4. Regex用于C#中的电子邮件验证
为了验证多个电子邮件,我们可以使用以下正则表达式。我们用分隔符';'分隔电子邮件
^((w+([-+.]w+)*@w+([-.]w+)*.w+([-.]w+)*)s*[;]{0,1}s*)+$
如果你想使用分隔符',',那么使用这个
^((w+([-+.]w+)*@w+([-.]w+)*.w+([-.]w+)*)s*[,]{0,1}s*)+$
如果你想同时使用分隔符','和';',那么使用this
^((w+([-+.]w+)*@w+([-.]w+)*.w+([-.]w+)*)s*[;,.]{0,1}s*)+$
因此,通过使用上述正则表达式,您可以验证单个或多个电子邮件。
5. 用C#中的正则表达式验证用户输入
本文解释了如何使用正则表达式(System.Text. Regx类)。在C#和.NET中的正则表达式命名空间)。
我们可以使用正则表达式。匹配方法,接受输入和正则表达式并返回成功
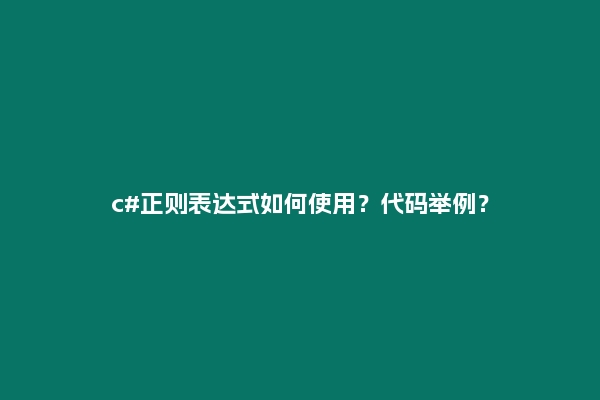
if (!Regex.Match(firstNameTextBox.Text, "^[A-Z][a-zA-Z]*$").Success) {}
if (!Regex.Match(addressTextBox.Text, @"^[0-9]+s+([a-zA-Z]+|[a-zA-Z]+s[a-zA-Z]+)$").Success)
if (!Regex.Match(cityTextBox.Text, @"^([a-zA-Z]+|[a-zA-Z]+s[a-zA-Z]+)$").Success)
if (!Regex.Match(stateTextBox.Text, @"^([a-zA-Z]+|[a-zA-Z]+s[a-zA-Z]+)$").Success)
if (!Regex.Match(zipCodeTextBox.Text, @"^d{5}$").Success)
{
if (!Regex.Match(phoneTextBox.Text, @"^[1-9]d{2}-[1-9]d{2}-d{4}$").Success)
6. 在C#中使用Regex.split拆分字符串
在这篇文章中,我们将学习如何在C#中使用Regex来分割字符串。
在这篇文章中,我们将学习如何在c#中使用RegEx来分割字符串。Regex根据模式拆分字符串。它处理指定为模式的分隔符。这就是为什么Regex优于string.Split。下面是一些如何在c#中使用Regex来分割字符串的例子。让我们开始编码。
为了便于使用,Regex添加了以下名称空间来分割字符串。
using System;
using System.Text.RegularExpressions;
using System.Collections.Generic;
示例1
使用正则表达式从字符串中分割出数字。
string Text = "1 One, 2 Two, 3 Three is good.";
string[] digits = Regex.Split(Text, @"D+");
foreach (string value in digits)
{
int number;
if (int.TryParse(value, out number))
{
Console.WriteLine(value);
}
}
上面的代码使用D+分割字符串,并通过check number和print循环。
7. 在C#中使用Regex替换字符串中的特殊字符
如果你有一个带有特殊字符的字符串,并想要删除/替换它们,那么你可以使用regex。
使用这段代码:
Regex.Replace(your String, @"[^0-9a-zA-Z]+", "")
这段代码将删除所有的特殊字符,但如果你不想删除一些特殊字符,例如逗号“,”和冒号“:”,那么做这样的更改:
Regex.Replace(Your String, @"[^0-9a-zA-Z:,]+", "")
同样,您也可以根据您的需求进行更改。
注意:
正则表达式并不是所有微小字符串都能解析的解决方案。如果您需要String类或其他类提供的简单解析,请尝试使用它们。
内容来自文章《C#正则表达式示例》,由“笨笨猿”作者发布于今日头条。